This project gave me my first taste of rendering. It began as an assignment for SFU’s “Introduction to Computer Graphics” course (CMPT 361); I was tasked with writing a basic software 3D renderer capable of performing Phong, Gouraud & flat shading over a span of 10 weeks.
I enjoyed this project so much that I decided to expand it by implementing ray-traced shadows & reflections similar to those described in Whitted’s 1980 paper An Improved Illumination Model for Shaded Display.
This program was written from scratch in C++. Use of external libraries was restricted to minor use of the C++ STL (Standard Template Library), and the QT framework for GUI windowing and pixel set/get operations. All other functionality was created specifically for this project.
GitHub Repository: https://github.com/b1skit/RayTracingRenderer
Sample Renders:
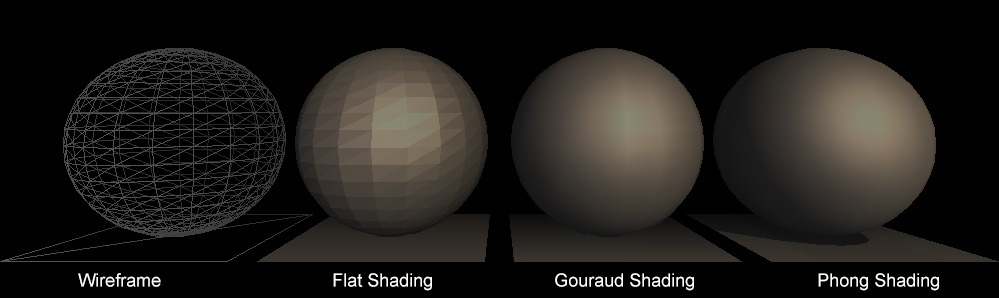
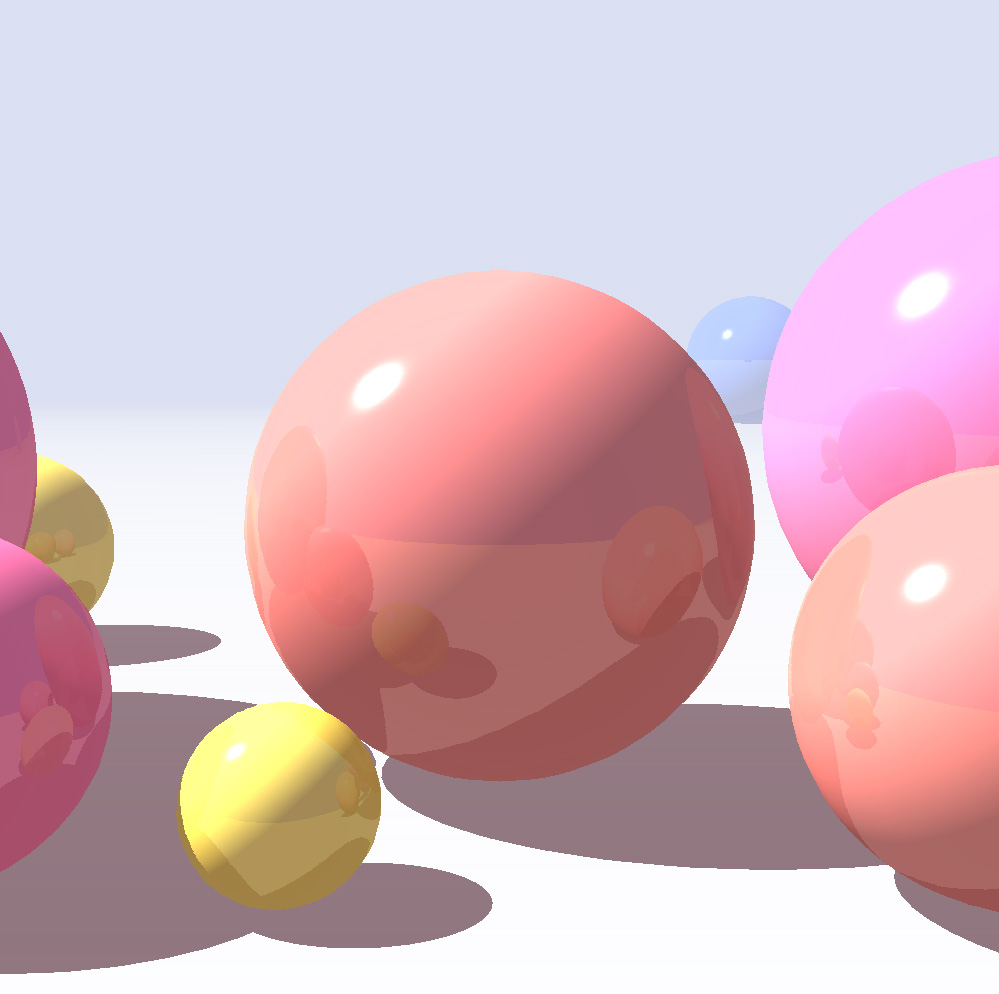
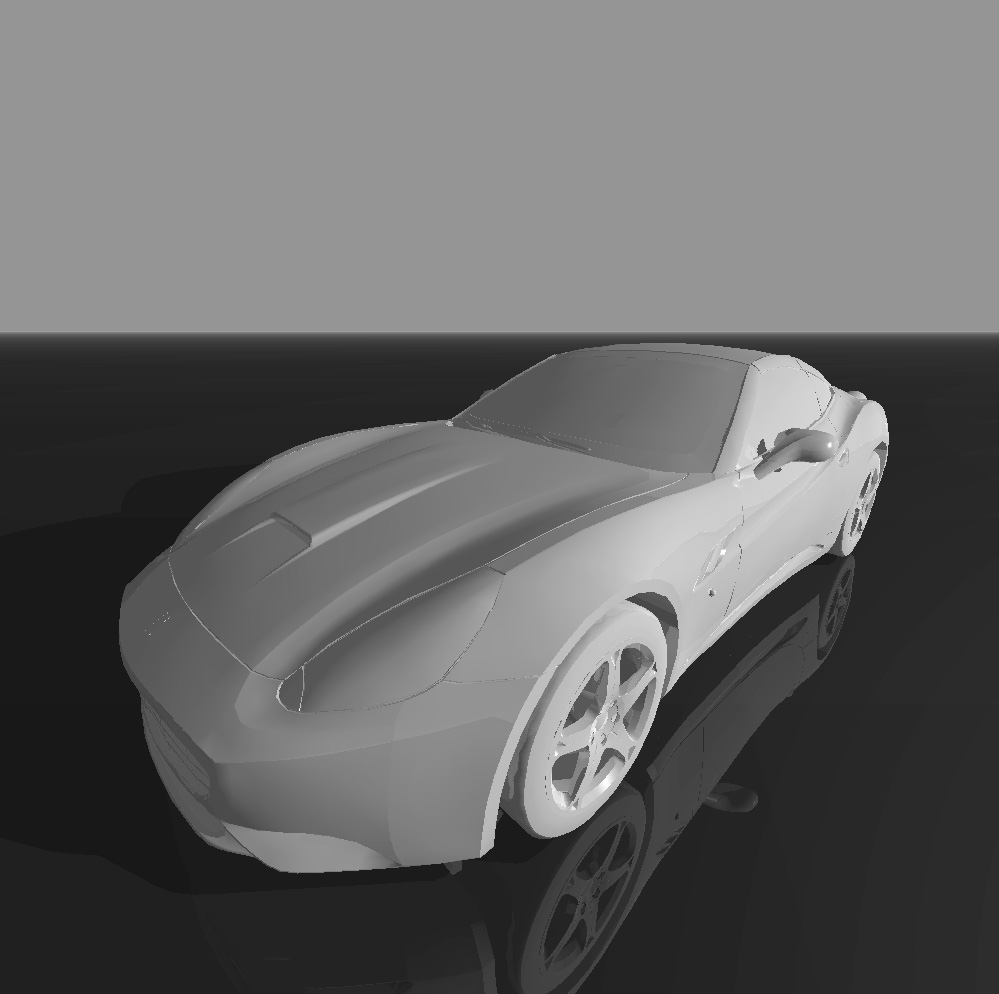
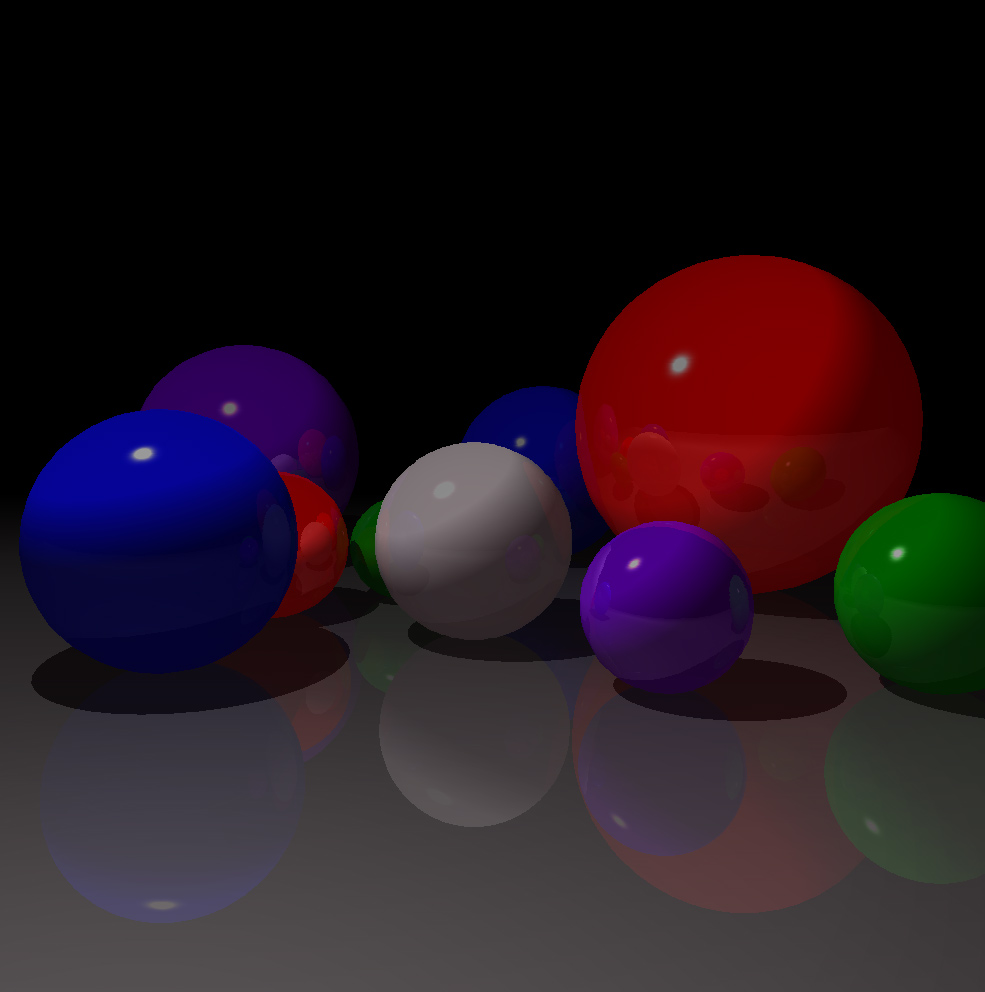
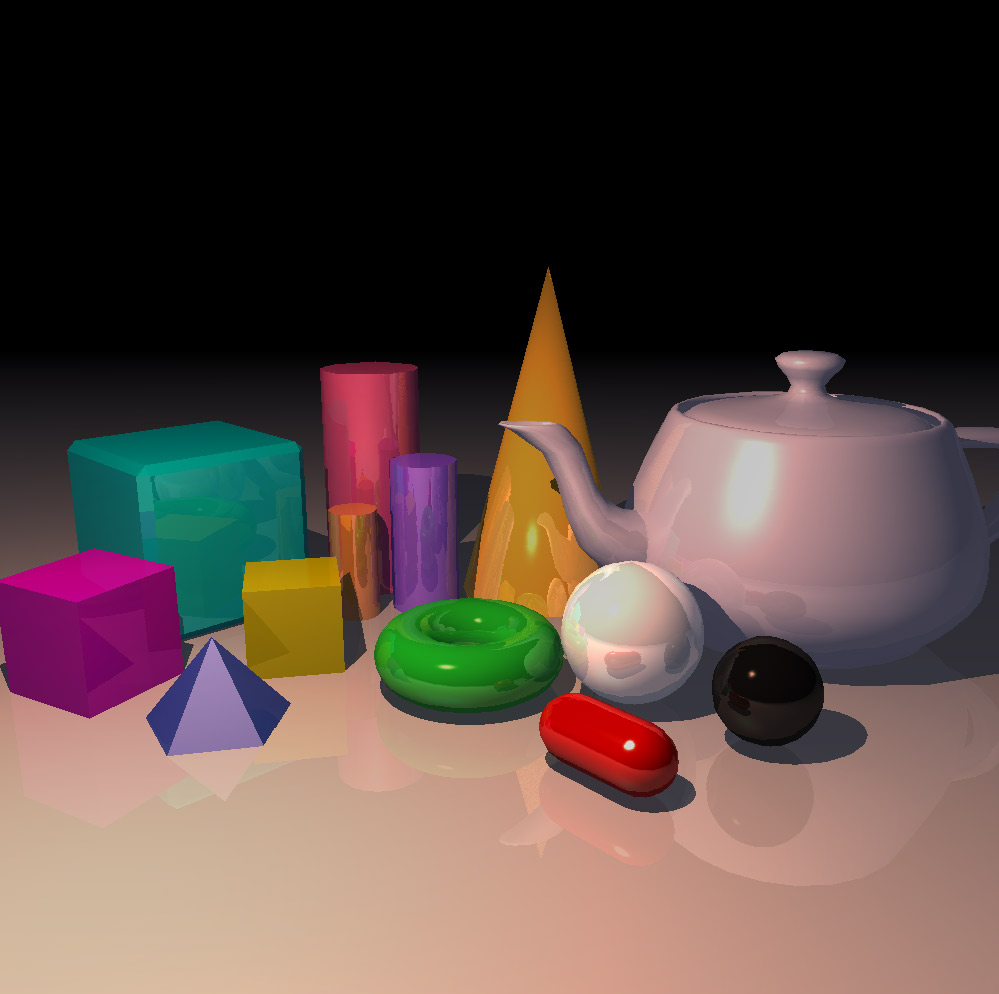
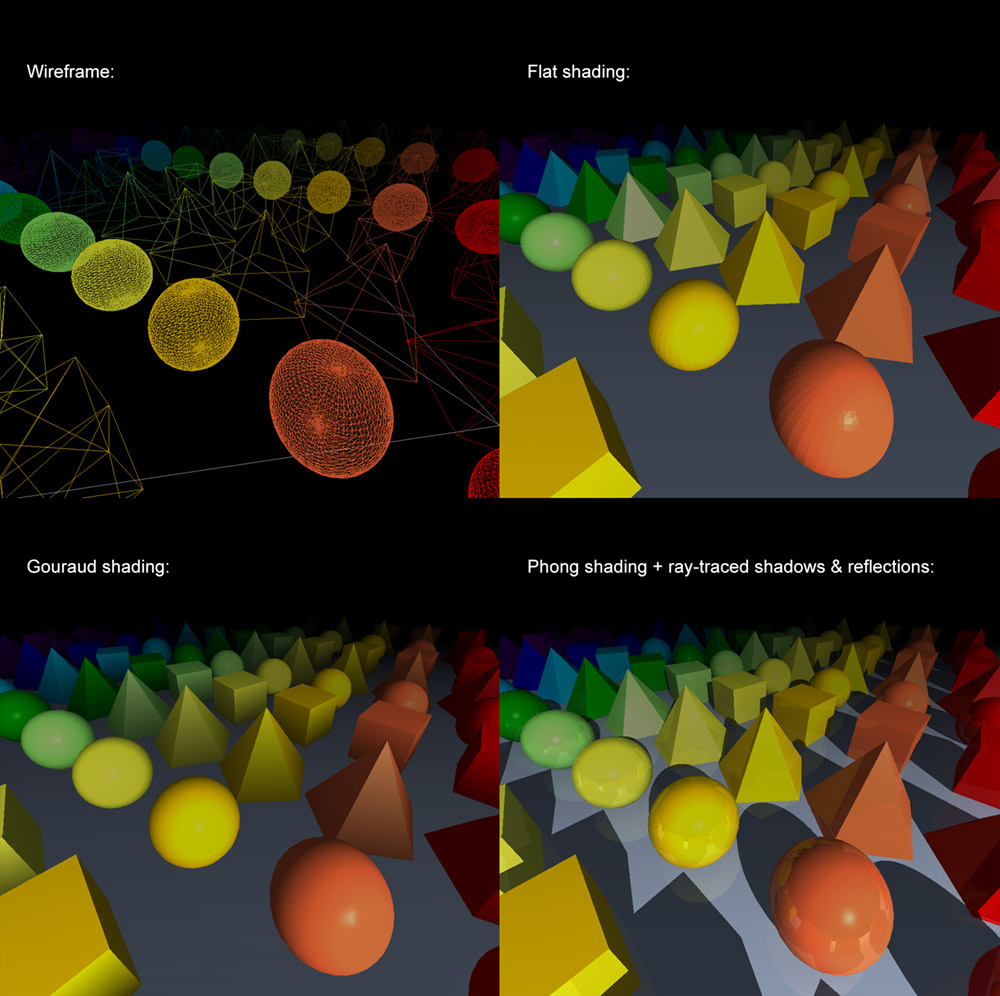
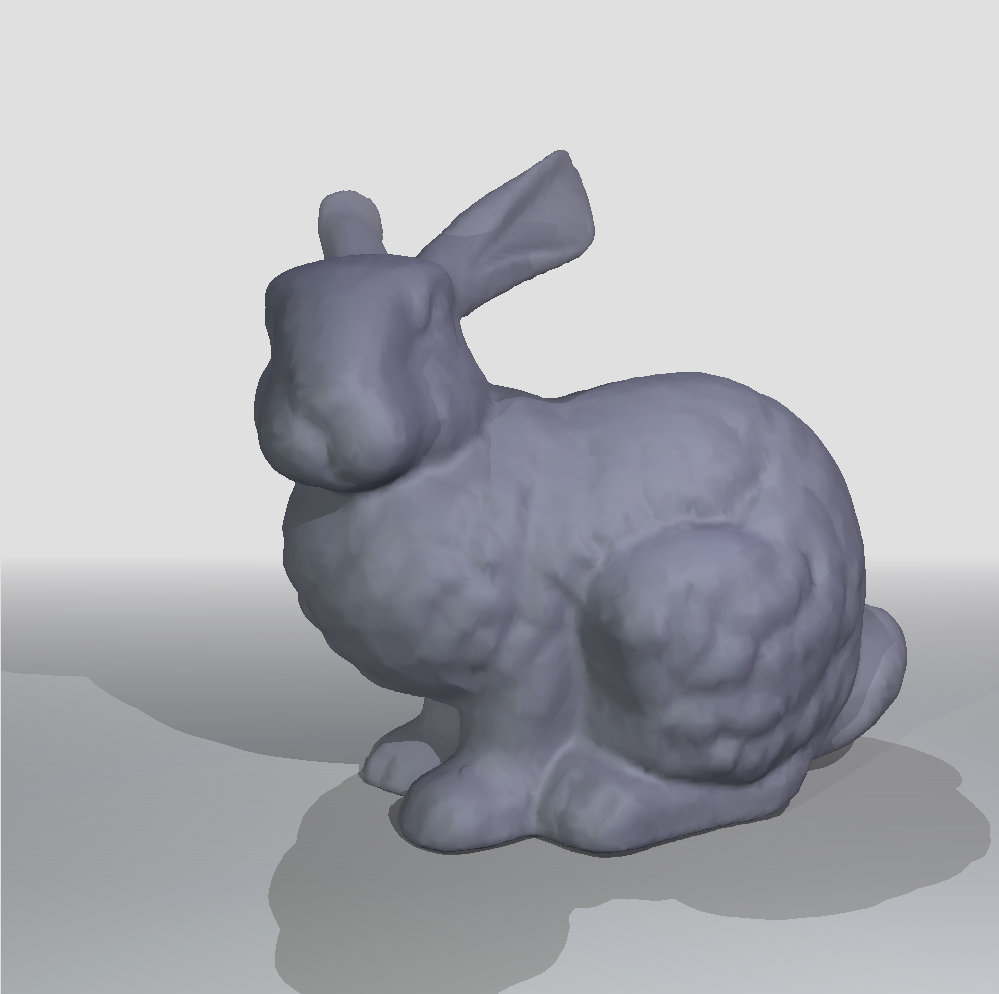
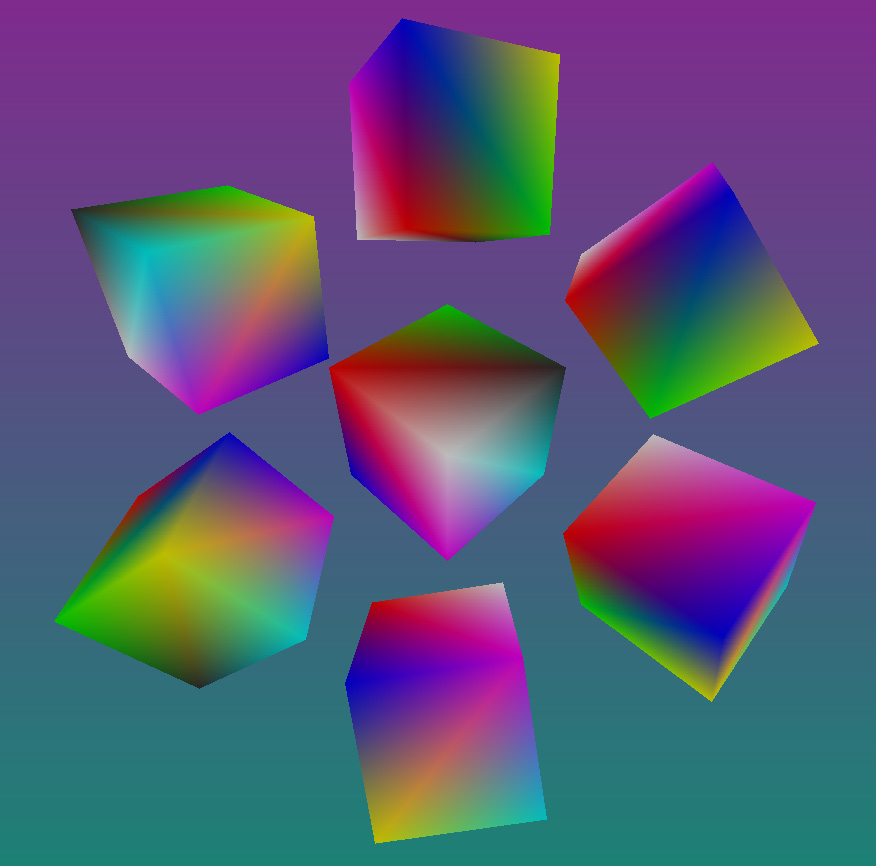
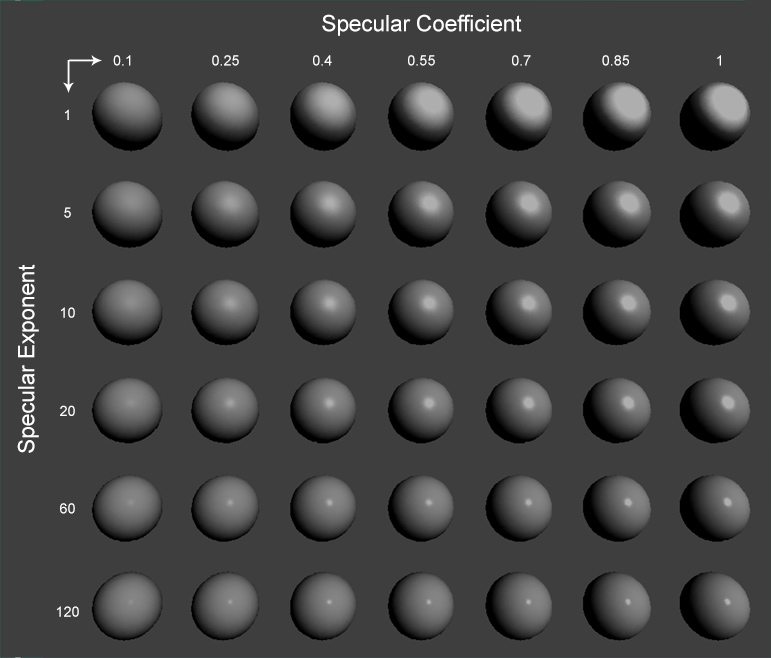
Renderer Features:
- Basic Rasterization:
- Triangle filling
- Line drawing
- Wireframe rendering
- Object transformations via matrices (Translation, rotation, scale of polygons, cameras & lights)
- Bi-linear interpolation (Perspective correct color & normal interpolation)
- Adjustable camera frustum/FOV (Field Of View) settings
- Shading Techniques:
- Flat/faceted shading (Lighting calculated once per face & applied to all vertices)
- Gouraud shading (Lighting calculated once per vertex & interpolated across faces)
- Phong shading (Per-pixel lighting)
- Lighting:
- Ray traced reflections & shadows
- Point lights (User-controlled world space position, color & attenuation)
- Ambient scene lighting
- Depth cued distance fog
- File Interpreter:
- Compatible with Wavefront 3D object format (.obj)
- 3D Scenes of can be fully constructed & controlled using a custom “simple” (.simp) ASCII file format
- Supports command line file rendering
- Render Pipeline Optimizations:
- Backface culling (Discards polygons not facing the camera)
- Frustum culling (Discards polygons outside of view frustum)
- Sutherland–Hodgman Polygon clipping (Removes portions of polygons outside of view frustum)
- n-gon Triangulation (Dividing convex polygons with 4 or more sides into triangles)
- Pixel depth/Z-Culling (Early-out if current point is behind the current raster Z buffer depth)
- Bounding boxes (Increased efficiency during ray intersection tests)